Debugging is an essential skill for developers, going beyond just fixing errors to understanding code deeply and improving reliability. It often takes more time than writing code itself, making efficient debugging techniques crucial. By mastering advanced tools and approaches, developers can save time, enhance problem-solving abilities, and write cleaner, more reliable code. This guide covers essential debugging strategies to help developers work smarter and solve issues effectively.
Understanding the debugging process
Effective debugging is not just trial and error – it’s a structured process that helps identify, isolate, and fix issues efficiently. The debugging process includes six key steps:
- Identify the Bug – Detect unexpected behavior or errors in the system.
- Reproduce the Bug – Ensure the issue can be consistently replicated to understand its cause.
- Diagnose the Root Cause – Use debugging tools, logs, or breakpoints to analyze and pinpoint the problem.
- Fix the Bug – Modify the code appropriately to resolve the issue.
- Test the Fix – Verify that the fix works correctly and does not introduce new problems.
- Prevent Future Bugs – Implement best practices such as writing test cases, improving logging, and following coding standards.
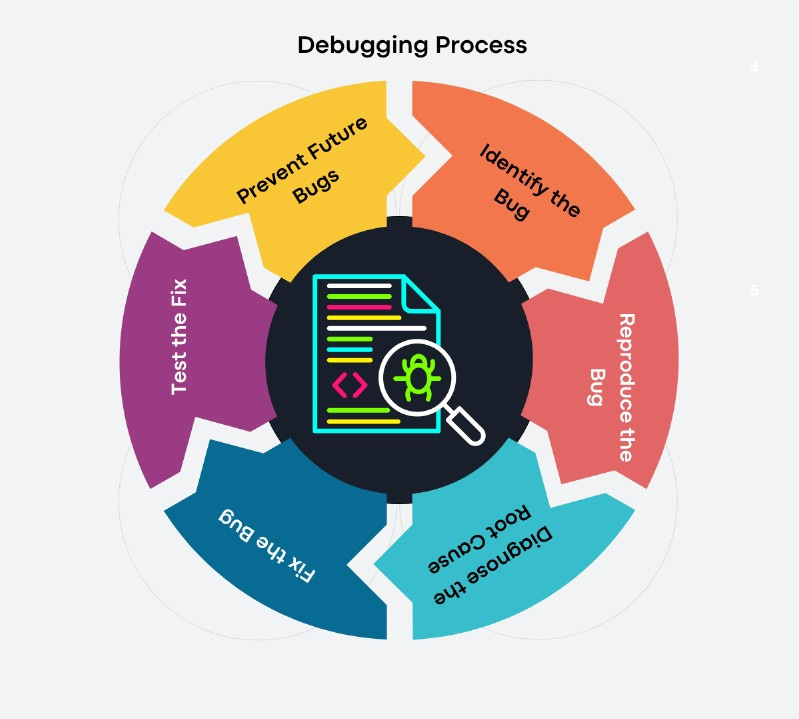
Essential Debugging tools
Debugging tools play a vital role in software development, helping developers find and fix errors quickly. As software systems become more complex, advanced debugging solutions are becoming more important. Many companies are investing in AI-powered debugging tools, and researchers are working on automated solutions to make the process even easier.
Using the right debugging tools can save time, improve efficiency, and help create more reliable applications. Whether you’re fixing a small bug or troubleshooting a major issue, having the right tools makes debugging faster and more effective.
- IDE Debuggers: Integrated Development Environments (IDEs) like Visual Studio Code, IntelliJ IDEA, Eclipse, and Xcode offer powerful debugging features. These include breakpoints to pause execution, step execution to navigate code line by line, and variable inspection to monitor values in real-time. Call stack analysis helps trace function calls, while conditional breakpoints stop execution only when specific conditions are met. These tools make debugging more efficient and precise.
- Browser Developer Tools: Web developers use tools like Chrome DevTools and Firefox DevTools to debug front-end issues, inspect HTML/CSS, analyze JavaScript performance, and track network activity.
- Logging and Monitoring Tools: Logging frameworks like Logstash, Kibana, Datadog, and Sentry help developers monitor system behavior and diagnose errors efficiently. They allow logs to be categorized by severity levels such as debug, info, warning, and error, making it easier to prioritize issues. These tools also enable the collection and analysis of logs across multiple services, helping detect and resolve production issues in real time, ensuring smoother application performance.
- Command-Line Debuggers: For backend and system debugging, command-line tools like GDB, LLDB, and PDB (Python Debugger) allow developers to inspect memory, step through execution, and analyze runtime behavior.
- API Debugging Tools: When testing APIs, tools like Postman and Insomnia help developers send test requests, analyze responses, and debug communication between client and server applications.
- Network Debugging Tools: For debugging network and distributed applications, tools like Wireshark, Charles Proxy, and Fiddler help monitor HTTP/HTTPS traffic and analyze request/response data.
- Specialized Debugging Tools: Certain languages have their own debugging tools:
- JavaScript: Chrome DevTools, React DevTools, Redux DevTools.
- Python: PDB, ipdb, PySnooper.
- Java: JConsole, VisualVM, Java Mission Control.
- Database Debugging: Query analyzers, database profilers.
- Command-Line Utilities: For system and server-side debugging, command-line tools like strace, ltrace, tcpdump, and top/htop help track system calls, monitor resource usage, and analyze network packets.
- Version Control Debugging: Version control systems like Git offer debugging capabilities:
- Git Bisect: Helps find which commit introduced a bug.
- Git Blame: Identifies who modified a line of code.
- Diffing Tools: Compare code versions to spot errors.
Effective debugging techniques
Effective debugging is a critical skill for developers, enabling them to identify and resolve issues efficiently. Here are some key techniques to enhance your debugging process:
- Understand the Problem: Before diving into the code, take time to comprehend the issue fully. Reproduce the bug consistently to observe its behavior and gather relevant information.
- Backtracing: Work backward from the point of failure to trace the origin of the problem. Reviewing recent code changes can often reveal the source of the issue.
- Utilize Debugging Tools: Leverage the debugging features of your Integrated Development Environment (IDE), such as setting breakpoints, stepping through code, and inspecting variables. These tools provide real-time insights into code execution.
- Set Breakpoints: Use breakpoints to pause code execution at specific points, allowing you to examine the program’s state and identify anomalies.
- Binary Search Method: When dealing with large codebases, apply the binary search technique by dividing the code into sections and testing each part to isolate the problematic area efficiently.
- Rubber Duck Debugging: Explain your code and the issue at hand to an inanimate object or a colleague. Articulating the problem often leads to new insights and solutions.
- Log Analysis: Implement logging to record the application’s runtime behavior. Analyzing logs helps in understanding the sequence of events leading to the bug and is invaluable for troubleshooting, especially in production environments.
- Clustering Bugs: Group similar bugs together to identify common patterns or root causes. This approach can lead to more effective and comprehensive fixes.
- Take Breaks: Stepping away from the problem can provide a fresh perspective. Short breaks often lead to breakthroughs that aren’t apparent when continuously focusing on the issue.
- Learn from Each Session: After resolving a bug, reflect on the debugging process. Documenting lessons learned can improve future debugging efficiency and prevent similar issues.
Debugging best practices
Debugging is an essential skill for developers, enabling them to identify and fix issues efficiently. Whether working with front-end interfaces, back-end services, or system-level code, understanding debugging tools and best practices enhances productivity.
1. Rule Debugging: Use console logging carefully, especially in platforms like Auth0. Avoid logging sensitive information. Tools like Real-time Webtask Logs help display logs in real-time.
2. Enable and Disable Debug Logging: Excessive logging can reduce performance. Use environment variables to control debug logging levels dynamically.
3. Static Analysis: Use tools like JSHint, SonarJS, and Coverity for static code analysis to detect vulnerabilities, loop detection, and overwrite detection.
Good Habits
1. Understand the Problem First: Before writing a single line of code, fully understand the issue. Gather information, reproduce the bug, and analyze the root cause.
2. Read and Interpret Error Messages: Error messages are roadmaps. Read them carefully, understand their meaning, and use them as a guide for debugging.
3. Write Testable Code: The easier your code is to test, the easier it is to debug. Follow best practices like modularity and single responsibility.
4. Collaborate: Don’t hesitate to ask for help but come prepared. Share what you’ve tried and what you’ve learned so far.
5. Prevent Bugs: The best way to debug is to avoid bugs altogether. Use code reviews, static analysis tools, and automated testing to catch issues early.
6. Stay Calm and Persistent: Debugging can be frustrating, but pros stay calm and methodical. They approach problems with patience and persistence, knowing that every bug is solvable with the right mindset.
Conclusion
Mastering debugging is a crucial skill for every developer, enabling them to solve issues efficiently, improve code quality, and enhance application reliability. By following a structured debugging process, leveraging powerful tools, and applying effective techniques, developers can streamline their workflows and reduce downtime caused by errors. Additionally, adopting best practices such as writing testable code, analyzing logs, and collaborating with others helps prevent future bugs and fosters continuous improvement. Debugging is not just about fixing problems—it’s about understanding the system, learning from mistakes, and refining coding skills. With the right mindset and approach, developers can turn debugging from a frustrating challenge into a valuable learning experience that strengthens their expertise in software development.